In this article, we will go over how to nest a sequence using ExtendScript in Adobe Premiere Pro. While the Premiere Pro scripting API does not natively offer a “nest” function, you can still use ExtendScript to accomplish this task by writing your own function to do so. In this example we will make use of the createSubsequence() method to mimic the nest functionality.
Nesting a sequence in Premiere Pro refers to taking a portion of your timeline and turning it into a new sequence. This new sequence can then be inserted back into the original sequence, making it a nested sequence.
Now, let’s take a look at a code example that will allow us to nest a sequence in Premiere Pro. We’ll write a function called nestSequence() which should nest the current selection.
In the screenshot below you can see the nestSelection() function that we will create as a part of this tutorial beeing executed in the ExtendScript Developer Tools. As you can see, the selected clip has been nested after executing the nestSelection() function.
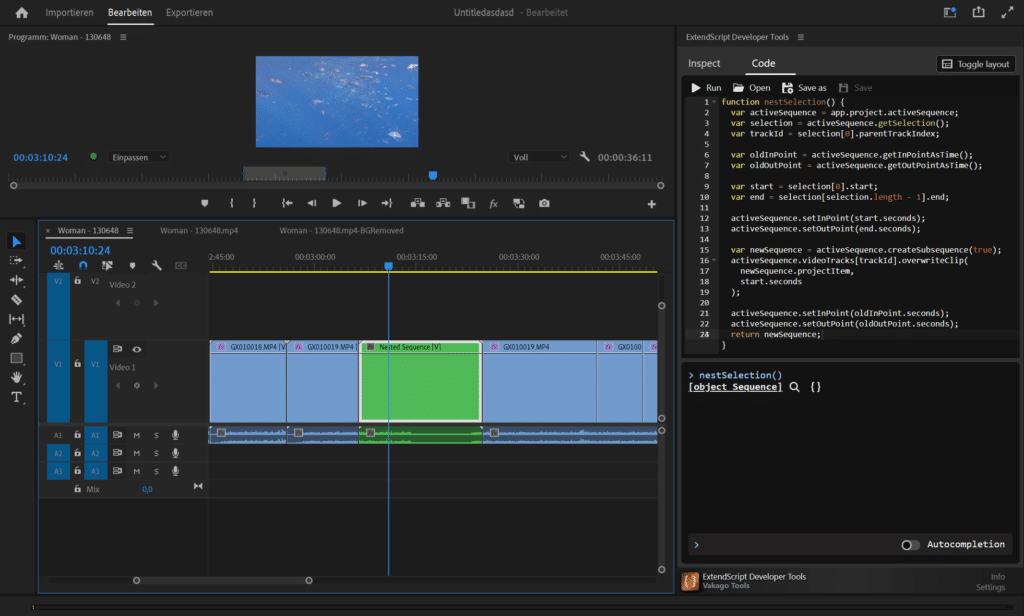
The first step is to get the active sequence in the project using app.project.activeSequence. Next, we get the current selection in the active sequence using the getSelection() method. Next, we get the track index of the first clip in the selection. This will be used to determine which track the new nested sequence should be inserted into.
As we’ll have to change the in / out point when creating a subsequence, we then store the current inPoint and outPoint of the active sequence, so that we can restore them later. The next step is to get the start and end times of the selection, which will be used to set the inPoint and outPoint of the active sequence.
Next, we create a new subsequence from the active sequence using the createSubsequence() method. Finally, we overwrite the the previously selected clip with the newly created sequence.
function nestSelection() {
var activeSequence = app.project.activeSequence;
// Get the current selection in the active sequence
var selection = activeSequence.getSelection();
if (selection.length < 1) {
return;
}
// We'll put the the sequence on the same track as the first item in the selection. The "cleaner" solution would be checking whether all clips are on the same track.
var trackId = selection[0].parentTrackIndex;
// In order to restore the previous in / out point we'll store the current values
var oldInPoint = activeSequence.getInPointAsTime();
var oldOutPoint = activeSequence.getOutPointAsTime();
// Get the start and end times of the selection
var start = selection[0].start;
var end = selection[selection.length - 1].end;
// Set the inPoint and outPoint of the active sequence to the start and end of the selection
activeSequence.setInPoint(start.seconds);
activeSequence.setOutPoint(end.seconds);
// Create a new subsequence from the active sequence
var newSequence = activeSequence.createSubsequence(true);
// Overwrite the first video track of the active sequence with the new subsequence
activeSequence.videoTracks[trackId].overwriteClip(
newSequence.projectItem,
start.seconds
);
// Restore the original inPoint and outPoint of the active sequence
activeSequence.setInPoint(oldInPoint.seconds);
activeSequence.setOutPoint(oldOutPoint.seconds);
// Return newly created sequence
return newSequence;
}
nestSelection();
ExtendScript Developer Tools
This article deals with the ExtendScript Developer Tools plugin for the Adobe Creative Cloud applications. Use below buttons to learn more.
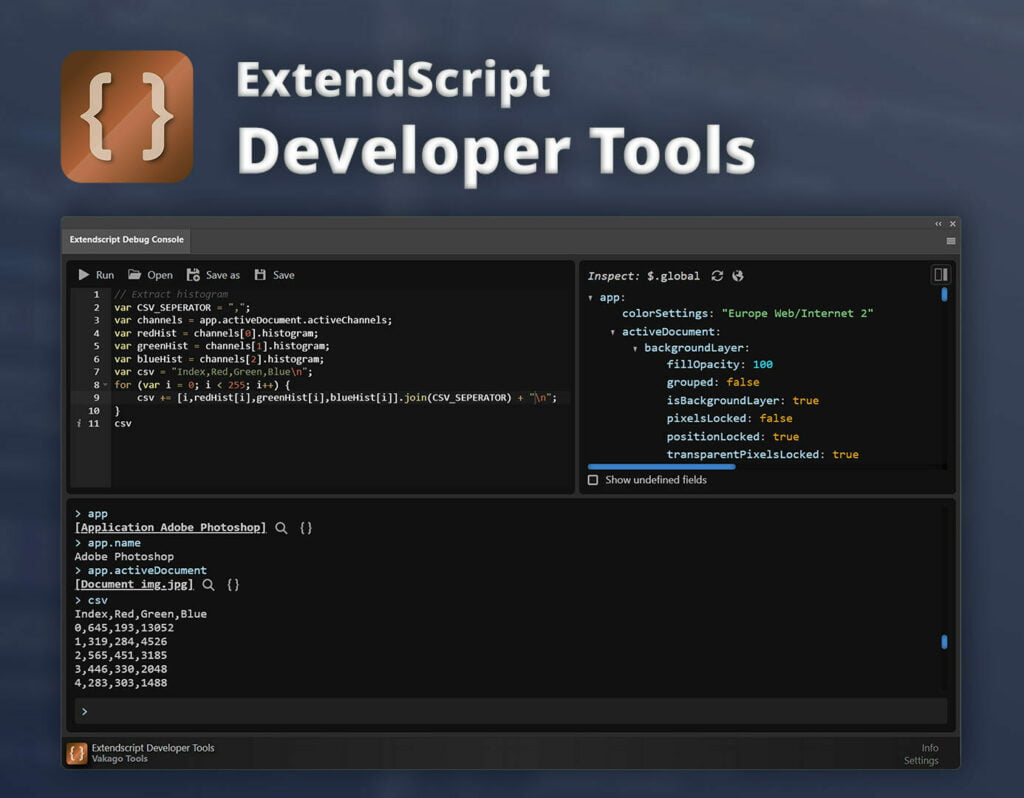