While it is easy to get the frame rate of a sequence in Premiere Pro using ExtendScript, getting the original frame rate of a clip is not trivial. However, by using the XMP scripting API, you can retrieve the frame rate of a clip or any other project item.
The XMP scripting API provides access to metadata associated with media files, including the frame rate of a clip. First, you need to load the AdobeXMPScript library. You can load it using the following code:
if (ExternalObject.AdobeXMPScript == undefined ) {
ExternalObject.AdobeXMPScript = new ExternalObject("lib:AdobeXMPScript");
}
Next, we’ll create a method that returns the framerate for a clip (TrackItem). The getFrameRate() function first creates an XMP metadata object using the getProjectMetadata() method of the projectItem object associated with the clip. It then checks if the Column.Intrinsic.MediaTimebase property exists in the PPRO_PRIVATE_NS namespace. If the property exists, it retrieves the frame rate and returns it as a float value. Keep in mind, that based on your locale the Framerate might not use a decimal separator that is not recognized by the parseFloat() function.
var PPRO_PRIVATE_NS = "http://ns.adobe.com/premierePrivateProjectMetaData/1.0/";
function getFrameRate(clip) {
var pxmp = new XMPMeta(clip.projectItem.getProjectMetadata())
if (pxmp.doesPropertyExist(PPRO_PRIVATE_NS, 'Column.Intrinsic.MediaTimebase') == true) {
frameRate = pxmp.getProperty(PPRO_PRIVATE_NS, 'Column.Intrinsic.MediaTimebase')
frameRate = parseFloat(frameRate)
return frameRate;
}
}
ExtendScript Developer Tools
Easily debug and test your ExtendScript code within the ExtendScript Developer Tools’ user-friendly interface, ensuring that your code runs smoothly before implementing it into your project. With features like auto completion and object inspection, you can quickly identify and resolve any issues in your code, improving your workflow and efficiency.
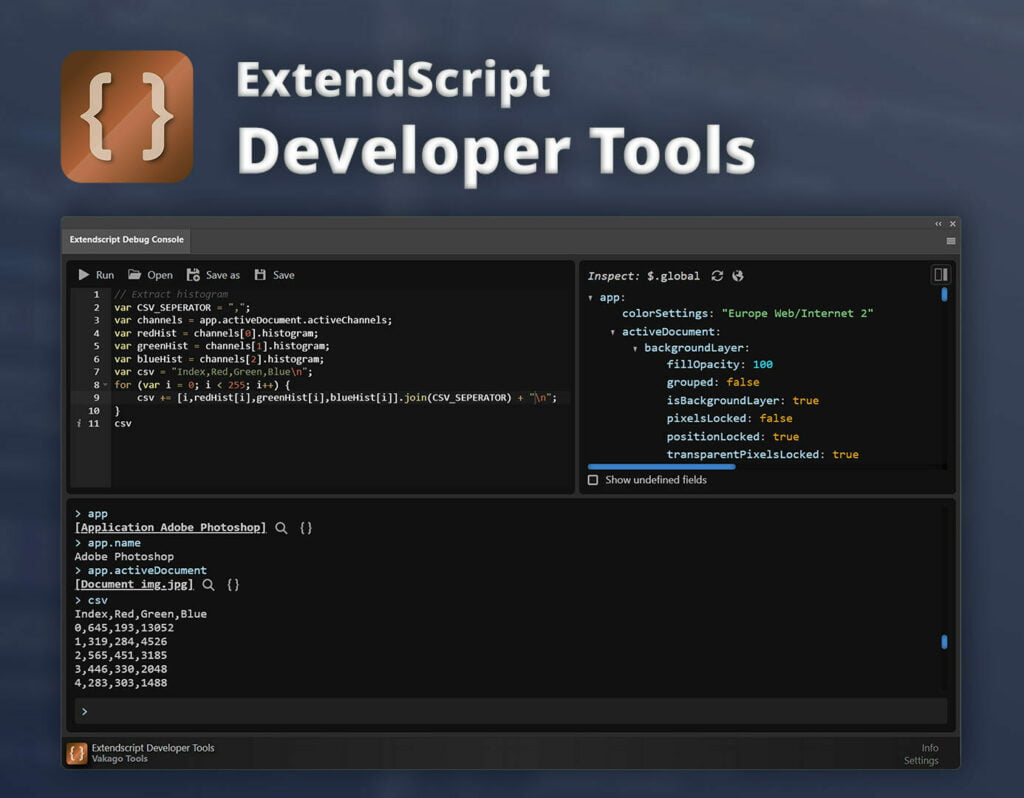
Next, we’ll test this function by testing it on the selected clip. You can retrieve the selected clip in the Premiere Pro timeline using the getSelection() method of the activeSequence object
var clip = app.project.activeSequence.getSelection()[0];
var frameRate = getFrameRate(clip);
To verify that the code for retrieving the frame rate of a Premiere Pro clip using ExtendScript works correctly, you can use the ExtendScript Developer Tools. Upon testing, the code returned the expected frame rate of 60fps, as shown in the screenshot below:
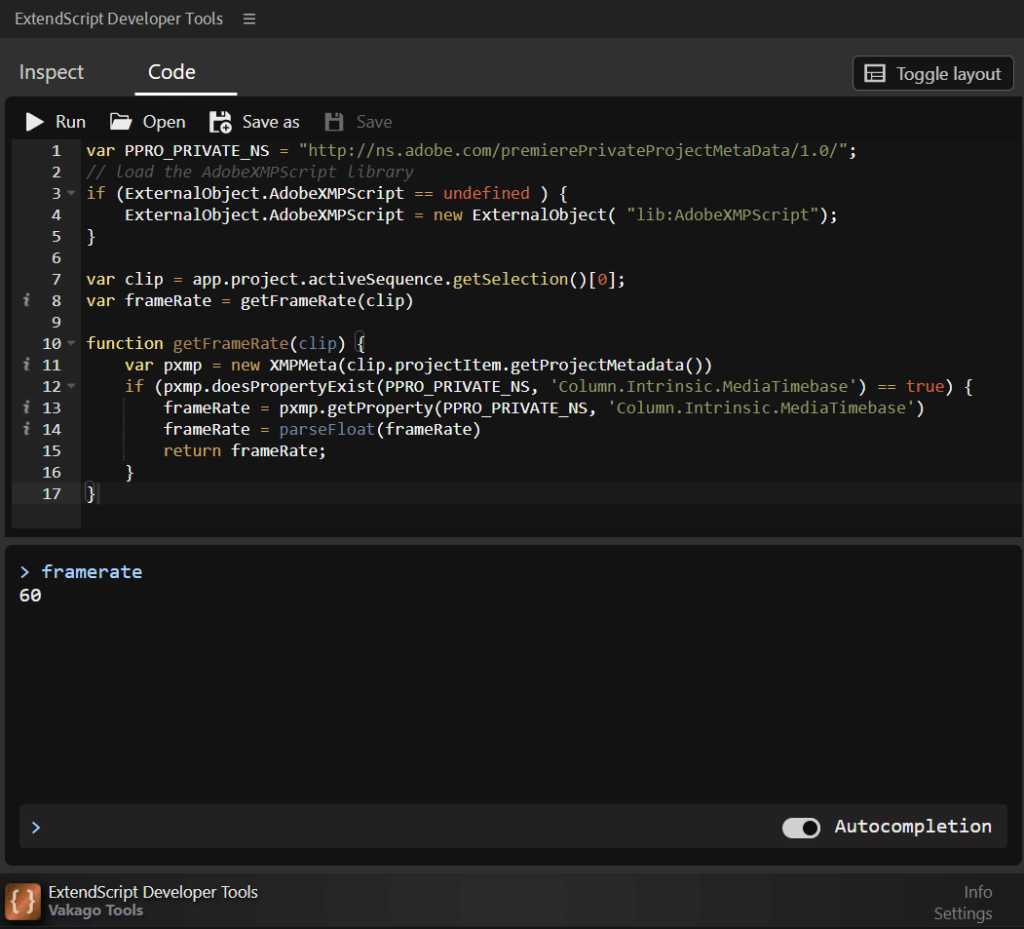
Screenshot showing the ExtendScript Developer Tools,
showing that the code correctly retrieved the frame rate of a Premiere Pro clip as 60fps.
Related Articles:
- How to Assign Shortcuts to ExtendScript
- Premiere Pro TRUE Fullscreen Tutorial: Hide Title, Header and Status Bar
- ExtendScript Tutorial: Adding Effect to a Clip in Premiere Pro
- Retrieving the Frame Rate of a Premiere Pro Clip using ExtendScript
- How To: Batch Update Links in Adobe Illustrator
- Tutorial: Premiere Pro Automation
- How to Nest a Sequence using ExtendScript in Premiere Pro
- Develop Your First Premiere Pro Extension: A Step-by-Step Guide
- Understanding the Adobe Premiere Pro QE API
- How to show a File Open / File Close Dialog in Adobe CEP